Installation :
install from nuget package manager (check for latest version while installing):
PM > Install-Package LazZiya.ImageResize
Introduction
All ImageResize methods are extensions to System.Drawing.Image
, so all resizing operations can be chained together.
System.Drawing.Image
can be created from local file as below :
using System.Drawing;
//create Image file from local file
var imgFile = Image.FromFile("wwwroot\\Images\\picture.jpg");
or from upload stream :
using System.Drawing;
//create Image file from upload stream
foreach (var file in Request.Form.Files) {
if (file.Length > 0) {
using (var stream = file.OpenReadStream()) {
var uploadedImage = Image.FromStream(stream);
}
}
}
Sample image for demo
For all below samples I used a picture taken by me in Istanbul :) click below to see the original file in a new tab:
Dimentions: 9598 x 3662
Size: 7.23 MB
Image properties
ImageResize
supports resizing images in several ways, all scale methods will keep the aspect ratio of the original image, except Crop method which will have a new aspect ratio according to the crop size.;
Scale by Width
TopScale down by given width parameter and keep original aspect ratio
using System.Drawing;
using LazZiya.ImageResize;
// use using to dispose the image after resize and free memory
using (var img = Image.FromFile(@"wwwroot\images\istanbul-panoramic.jpg"))
{
img.ScaleByWidth(600)
.SaveAs(@"wwwroot\images\new-istanbul-panoramic.jpg");
}
Result image size 600x228
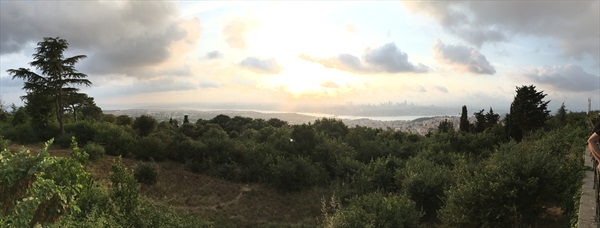
Scale by Height
TopScale down by given height parameter and keep original aspect ratio
using (var img = Image.FromFile(@"wwwroot\images\istanbul-panoramic.jpg"))
{
img.ScaleByHeight(300)
.SaveAs(@"wwwroot\images\H300-istanbul-panoramic.jpg");
}
Result image size: 786x300
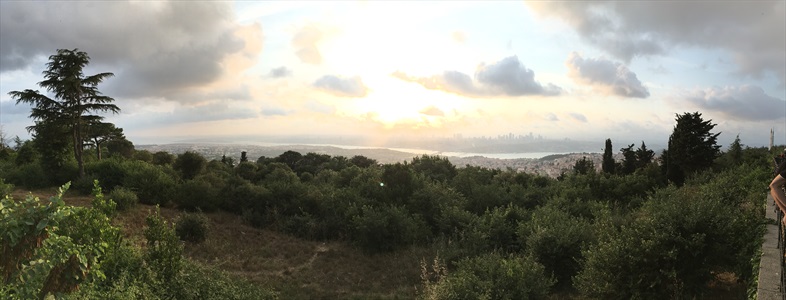
Scale
TopAuto scale down image till longest border is equal to the target dimension and keep original aspect ratio
using (var img = Image.FromFile(@"wwwroot\images\istanbul-panoramic.jpg"))
{
img.Scale(600, 400)
.SaveAs(@"wwwroot\images\scale-istanbul-panoramic.jpg");
}
Result image size: 600x228
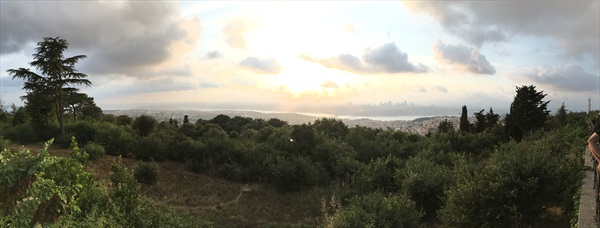
Scale and crop
TopScale down by width and height till shortest border is equal to the requested value, then crop the extras. Final image will have the exact given new width and height but could have different aspect ratio depending on new dimensions.
using (var img = Image.FromFile(@"wwwroot\images\istanbul-panoramic.jpg"))
{
img.ScaleAndCrop(600, 400)
.SaveAs(@"wwwroot\images\scale-istanbul-panoramic.jpg");
}
Result image size: 600x400
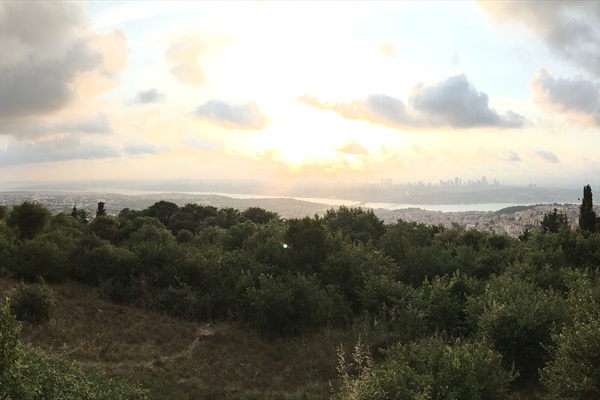
Scale and crop target spot
TopBy default ScaleAndCrop will target the image center. Additionally it is possible to specify different area of the image to be scaled/cropped and generate the final result. You can choose from pre-defined 9 spots (3 rows, 3 columns) using TargetSpot attribute
using (var img = Image.FromFile(@"wwwroot\images\istanbul-panoramic.jpg"))
{
img.ScaleAndCrop(600, 400, TargetSpot.MiddleRight)
.SaveAs(@"wwwroot\images\scale-crop-middle-right-istanbul-panoramic.jpg");
}
Result image size: 600x400
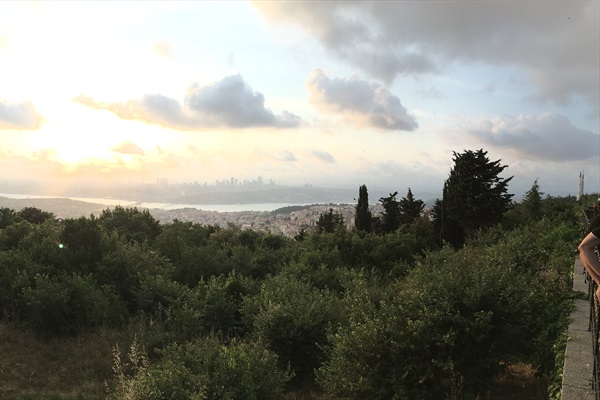
Crop
TopCrop the image by given sizes.
using (var img = Image.FromFile(@"wwwroot\images\istanbul-panoramic.jpg"))
{
img.Crop(600, 600)
.SaveAs(@"wwwroot\images\crop-istanbul-panoramic.jpg");
}
Result image size: 600x600
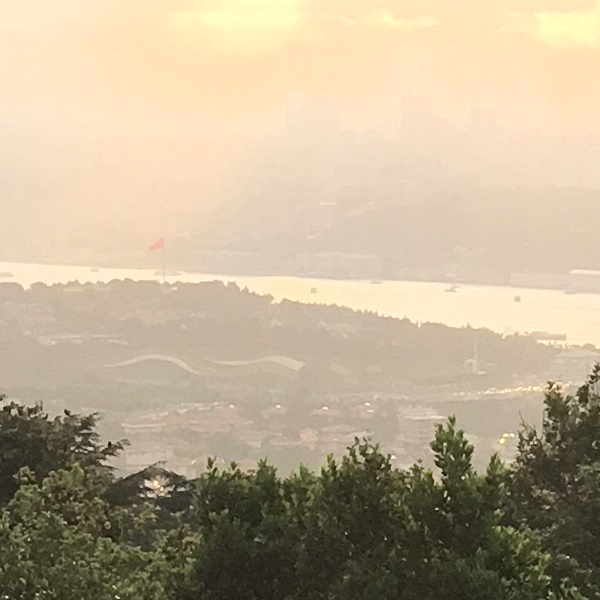
Crop target spot
TopCrop specified spot from the the image by given sizes. By default image center will be cropped, but it is possible to target different area of the image to be cropped:
using (var img = Image.FromFile(@"wwwroot\images\istanbul-panoramic.jpg"))
{
img.Crop(600, 600, TargetSpot.BottomRight)
.SaveAs(@"wwwroot\images\crop-bottom-right-istanbul-panoramic.jpg");
}
Result image size: 600x600
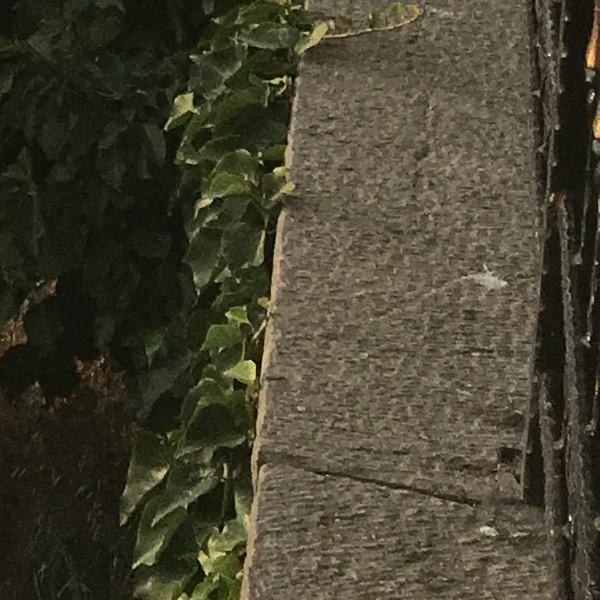
Text Watermark
TopAdd text watermark to the image. In below sample first I will resize the image then add text watermark to the resized image.
using (var img = Image.FromFile(@"wwwroot\images\istanbul-panoramic.jpg"))
{
img.Crop(500, 500)
.AddTextWatermark("http://demo.ziyad.info")
.SaveAs(@"wwwroot\images\text-watermark-istanbul-panoramic.jpg");
}
Result image size: 500x500
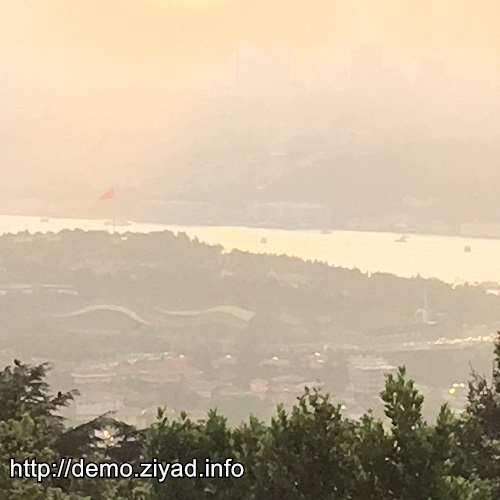
Text Watermark Options
TopUse TextWatermarkOptions to customize settings of the text watermark.
var twmOps = new TextWatermarkOptions
{
Location = TargetSpot.TopRight,
FontSize = 50,
FontName = "Edwardian Script ITC",
// Text with red color and half opacity
TextColor = Color.FromArgb(200, Color.Red),
// Use alpha channel to specify color opacity
// e.g. use 0 opacity to disable drawing the outline
OutlineColor = Color.FromArgb(0, Color.White),
};
using (var img = Image.FromFile(@"wwwroot\images\istanbul-panoramic.jpg"))
{
img.Crop(500, 500)
.AddTextWatermark("demo.ziyad.info", twmOps)
.SaveAs(@"wwwroot\images\text-watermark-ops-istanbul-panoramic.jpg");
}
Result image
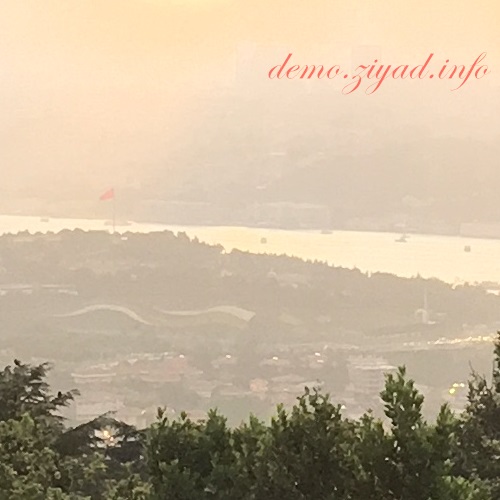
Image Watermark
TopDraw image watermark over the image. First we will resize the image to 500x500 then watermark will be applied.
using (var img = Image.FromFile(@"wwwroot\images\istanbul-panoramic.jpg"))
{
var iwm = Image.FromFile(@"wwwroot\images\wm.png");
img.Crop(500, 500)
.AddImageWatermark(iwm)
.SaveAs(@"wwwroot\images\image-watermark-istanbul-panoramic.jpg");
iwm.Dispose();
}
Result image
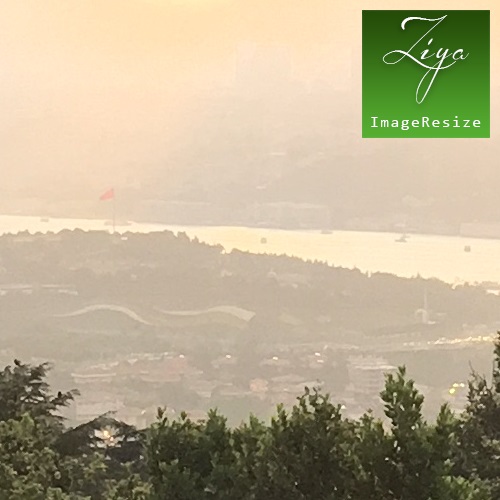
Image Watermark Opitons
TopUse ImageWatermarkOptions to customize settings of the image watermark.
var iwmOps = new ImageWatermarkOptions
{
Location = TargetSpot.BottomRight,
// 0 full transparent, 100 full color
Opacity = 50,
};
using (var img = Image.FromFile(@"wwwroot\images\istanbul-panoramic.jpg"))
{
var iwm = Image.FromFile(@"wwwroot\images\wm.png");
img.Crop(500, 500)
.AddImageWatermark(iwm, iwmOps)
.SaveAs(@"wwwroot\images\image-watermark-ops-istanbul-panoramic.jpg");
iwm.Dispose();
}
Result image
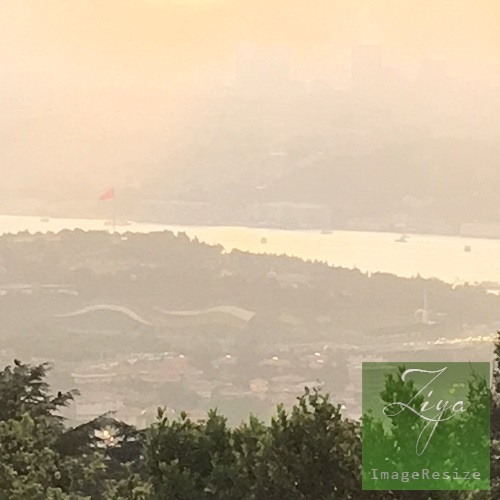
If you need to resize the watermark, use same pethods to resize it then save it to the disc and then use Image.FromFile as described above.
Combining resize settings
TopAs mentioned earlier, all methods can be chained together to provide easily image processing.
Additionally, LazZiya.ImageResize supports multilingual texts as shown below.
var twmOps = new TextWatermarkOptions
{
Location = TargetSpot.Center,
FontSize = 35,
FontStyle = FontStyle.Bold,
// Don't draw text
TextColor = Color.FromArgb(0, Color.White),
// Draw outline only, alpha (0 - 255)
OutlineColor = Color.FromArgb(125, Color.BlueViolet),
OutlineWidth = 2
};
var iwmOps = new ImageWatermarkOptions
{
Location = TargetSpot.BottomMiddle,
// 0 full transparent, 100 full color
Opacity = 30,
};
using (var img = Image.FromFile(@"wwwroot\images\istanbul-panoramic.jpg"))
{
var iwm = Image.FromFile(@"wwwroot\images\wm.png");
img.Crop(500, 500)
.AddImageWatermark(iwm, iwmOps)
.AddTextWatermark("Türkçe 中國 العربية", twmOps)
.SaveAs(@"wwwroot\images\image-text-istanbul-panoramic.jpg");
iwm.Dispose();
}
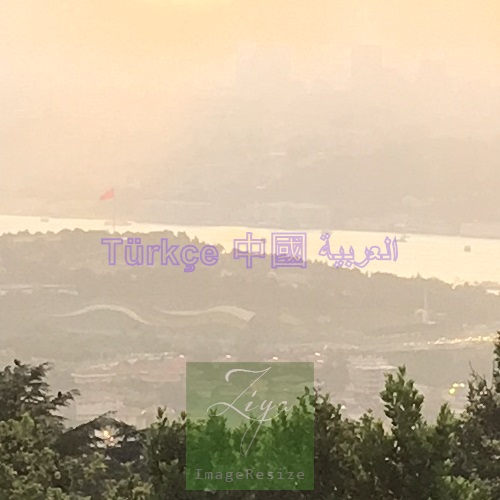